NumberOfDiscIntersections O(N) solution Task The task is to count overlapping discs given an array of radii We draw N discs on a plane. The discs are numbered from 0 to N − 1. An array A of N non-negative integers, specifying the radiuses of the discs, is given. The J-th disc is drawn with its […]
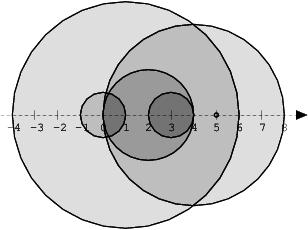